Welcome to the next chapter of our WordPress theme development journey! In this chappter, we explore how to add dynamic Widgets and Sidebars in WordPress. These tools offer countless options to enhance your theme’s functionality and tailor your website to your exact specifications.
Let’s dive into the process of registering widget areas, seamlessly adding widgets, and expanding your website with engaging, dynamic content.
Registering Widget Areas (Sidebars)
Before you can start adding widgets, you need a designated space for them. This is where widget areas, often referred to as sidebars, come into play. You can think of sidebars as predefined regions on your website where you can place widgets.
To register a widget area in your WordPress theme, you’ll typically need to modify your theme’s functions.php
file. Here’s an example of how to register a simple sidebar:
function theme_register_sidebar() {
register_sidebar(array(
'name' => __('Right Sidebar Widget', 'custom-theme'),
'id' => 'right-sidebar',
'description' => __('Add widgets here to display in the Sidebar Widget.', 'custom-theme'),
'before_widget' => '<div id="%1$s" class="widget %2$s">',
'after_widget' => '</div>',
'before_title' => '<h2 class="widget-title">',
'after_title' => '</h2>',
));
}
add_action('widgets_init', 'theme_register_sidebar');
In the code above, we’re registering a widget area named ‘Main Sidebar’ with an ID of ‘main-sidebar.’ You can customize the name, description, and appearance of your widget area as needed.
After adding above into functions.php, you should see widget added in Theme/Appearence
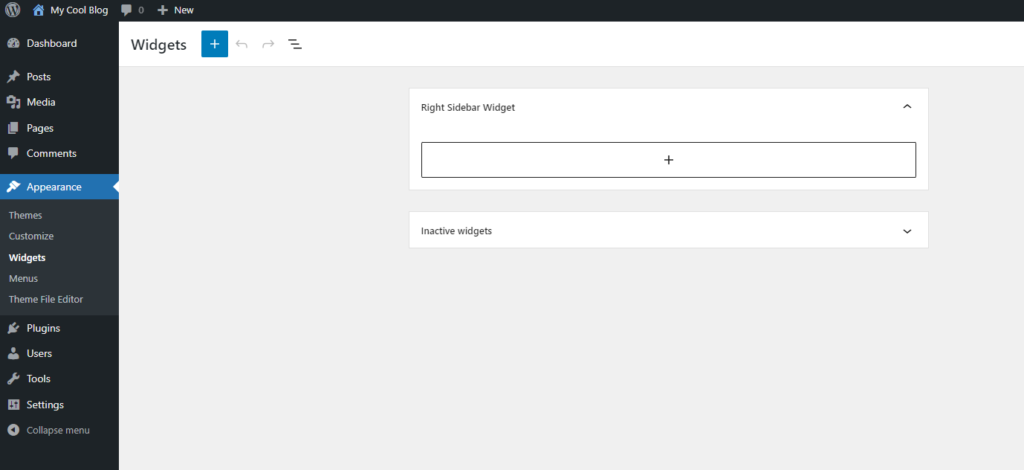
Adding Widgets to Widget Areas
Once your widget area is registered, you can start adding widgets to it. Widgets are modular pieces of content or functionality that can be easily dragged and dropped into your widget areas. WordPress comes with several built-in widgets like Recent Posts, Categories, and Search. You can also find many additional widgets in the WordPress Plugin Repository.
To add widgets to your sidebar, go to your WordPress admin dashboard, navigate to “Appearance” > “Widgets,” and you’ll see your registered widget area on the right-hand side. From there, you can simply drag and drop widgets into your widget area and configure their settings.
Customizing Widget Areas with Dynamic Content
What makes widgets and sidebars powerful is their ability to display dynamic content. This content can range from recent posts and popular tags to custom content like advertisements, newsletter sign-up forms, or social media feeds.
To customize your widget areas with dynamic content, you can use widgets like:
- Text Widget: This widget allows you to add custom HTML, text, or shortcodes. You can use it to embed videos, display ads, or create custom calls to action.
- Recent Posts Widget: It automatically displays a list of your most recent blog posts, keeping your visitors engaged with your latest content.
- Categories Widget: This widget lists the categories on your site, helping visitors explore your content by topic.
- Custom HTML Widget: If you have custom code or want to embed content from third-party services, this widget is perfect for the job.
- Social Media Widgets: Many themes come with built-in social media widgets that allow you to display your social feeds or links to your profiles.
By thoughtfully selecting and arranging widgets, you can craft a website that offers a rich and engaging experience for your visitors. This not only enhances your site’s functionality but also keeps your audience informed and entertained.
For the sake of demonstration, let’s add “recent posts” and “archives” widgets in our side bar.
Now we want to display our right side bar,
<?php if (get_theme_mod('show_right_sidebar', true)) { ?>
<?php if (is_active_sidebar('right-sidebar')) : ?>
<ul class="list-unstyled text-left home-right-sidebar">
<div id="sidebar-nav" class="border-0 rounded-0 text-sm-start min-vh-100">
<?php dynamic_sidebar('right-sidebar'); ?>
</div>
</ul>
<?php endif; ?>
<?php } ?>
Breaking Down the Code:
- Conditional Check: The code begins with a conditional check. It verifies if the theme customizer setting ‘show_right_sidebar’ is set to ‘true’ before proceeding to display the sidebar. This conditional check ensures that the sidebar is only shown if it’s enabled in the theme’s settings.
- Sidebar Activation Check: Within the first condition, we have another check,
is_active_sidebar('right-sidebar')
. This verifies if there are active widgets in the ‘right-sidebar’ widget area. It will not display sidebar if widget area is empty. This way, you don’t end up with an empty or redundant sidebar. - Displaying the Widgets: If both conditions are met, the sidebar is displayed. We use an unordered list (
<ul>
) with the ‘list-unstyled’ class for styling purposes. Inside this list, we create a container div with the ID ‘sidebar-nav’ for additional styling. Finally, we use thedynamic_sidebar()
function to output the widgets placed in the ‘right-sidebar’ widget area.
Following is full index.php
file,
<?php get_header(); ?>
<div class="container mt-2 mt-md-5">
<div class="row">
<div class="col-md-8">
<?php
$paged = (get_query_var('paged')) ? get_query_var('paged') : 1;
$args = array(
'posts_per_page' => 10,
'paged' => $paged
);
$query = new WP_Query($args);
?>
<?php if ($query->have_posts()) : ?>
<?php while ($query->have_posts()) : $query->the_post(); ?>
<article <?php post_class(''); ?>>
<div class="row">
<h2><a class="no-underline" href="<?php the_permalink(); ?>"><?php the_title(); ?></a></h2>
<div class="post-author-date">
<i class="fas fa-user"></i>
<span class="post-by">By <?php the_author(); ?></span>,
<i class="far fa-calendar"></i>
<span class="post-date"><?php the_date(); ?></span>
</div>
<div class="featured-image-home d-flex align-items-center justify-content-center">
<?php if (has_post_thumbnail()) : ?>
<a href="<?php the_permalink(); ?>"><?php the_post_thumbnail(); ?></a>
<?php endif; ?>
</div>
</div>
<div class="row">
<div class="home-post-excerpt pt-3">
<?php the_excerpt(); ?>
</div>
<div class="mt-2 d-flex justify-content-end">
<a href="<?php the_permalink(); ?>" class="btn btn-primary">Read More</a>
</div>
</div>
<div class="row mt-5"></div>
</article>
<? endwhile; ?>
<?php else : ?>
<p><?php _e('No posts found.', 'your-theme-text-domain'); ?></p>
<?php endif; ?>
<?php wp_reset_postdata(); ?>
</div>
<div class="col-md-3">
<?php if (get_theme_mod('show_right_sidebar', true)) { ?>
<?php if (is_active_sidebar('right-sidebar')) : ?>
<ul class="list-unstyled text-left home-right-sidebar">
<div id="sidebar-nav" class="border-0 rounded-0 text-sm-start min-vh-100">
<?php dynamic_sidebar('right-sidebar'); ?>
</div>
</ul>
<?php endif; ?>
<?php } ?>
</div>
</div>
</div>
<?php get_footer(); ?>
We should now side bar displayed,
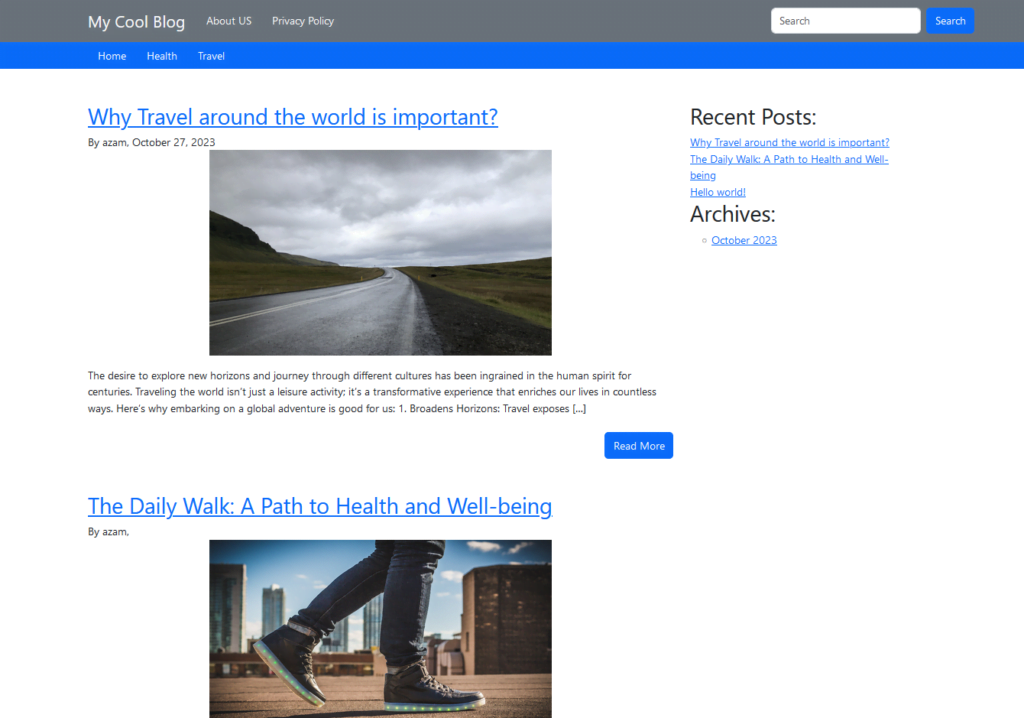
In this installment of our series, you’ve learned the essentials of registering widget areas, adding widgets to them, and infusing your theme with dynamic content. Widgets and sidebars are powerful tools that put you in control of your website’s appearance and functionality. Stay tuned for more insights and knowledge-sharing in our continuing journey through WordPress theme development.
Home: Creating WordPress Theme