Part 9: Adding Customized Theme Options of WordPress Theme
Welcome back to our WordPress theme development series. In this installment, we’re going to explore the exciting world of adding theme options to your theme. By using the powerful WordPress Customizer, we’ll create options for customizing colors, fonts, and more, allowing you to put a personal touch on your theme. Let’s dive in and see how it’s done.
Using the WordPress Customizer
The WordPress Customizer is a user-friendly tool that allows you to add a range of customization options to your theme, providing an intuitive interface for users to tailor the appearance of their websites. It’s where the magic happens when it comes to creating custom theme options.
To access the Customizer, you can navigate to your WordPress admin dashboard and go to “Appearance” > “Customize.” This will launch the Customizer, which typically includes a live preview of your website on the right side of the screen and a list of customizable options on the left.
Creating Theme Options for Colors, Fonts, and More
Now, let’s get to the fun part—creating custom theme options. To add options for colors, fonts, or any other aspect of your theme, you’ll need to modify your theme’s functions.php
file. Below is an example of how to create a custom theme option for changing the primary color of your theme using the WordPress Customizer:
function theme_customize_register($wp_customize) {
$wp_customize->add_section('theme_fonts', array(
'title' => __('Site Fonts', 'custom-theme'),
));
// Define an array of font options
$font_options = array(
'Source Sans Pro, sans-serif' => __('Source Sans Pro, sans-serif', 'custom-theme'),
'Arial, sans-serif' => __('Arial, sans-serif', 'custom-theme'),
'Georgia, serif' => __('Georgia, serif', 'custom-theme'),
'Tahoma, sans-serif' => __('Tahoma, sans-serif', 'custom-theme'),
'Roboto, sans-serif' => __('Roboto, sans-serif', 'custom-theme'),
'Open Sans, sans-serif' => __('Open Sans, sans-serif', 'custom-theme'),
);
$wp_customize->add_setting('default_text_font', array(
'default' => 'Roboto, sans-serif',
'transport' => 'refresh',
));
$wp_customize->add_control('default_text_font', array(
'label' => __('Text Font', 'custom-theme'),
'section' => 'theme_fonts',
'type' => 'select',
'choices' => $font_options,
));
$wp_customize->add_section('theme_colors', array(
'title' => __('Site Colors', 'custom-theme'),
));
$wp_customize->add_setting('topbar_color', array(
'default' => '#3388FF',
'sanitize_callback' => 'sanitize_hex_color',
));
$wp_customize->add_control(new WP_Customize_Color_Control($wp_customize, 'topbar_color', array(
'label' => __('Top bar Color', 'custom-theme'),
'section' => 'theme_colors',
)));
$wp_customize->add_setting('navigation_bar_color', array(
'default' => '#3388FF',
));
$wp_customize->add_control(new WP_Customize_Color_Control($wp_customize, 'navigation_bar_color', array(
'label' => __('Navigation Bar Color', 'custom-theme'),
'section' => 'theme_colors',
)));
$wp_customize->add_setting('button_color', array(
'default' => '#3388FF',
'sanitize_callback' => 'sanitize_hex_color',
));
$wp_customize->add_control(new WP_Customize_Color_Control($wp_customize, 'button_color', array(
'label' => __('Button Color', 'custom-theme'),
'section' => 'theme_colors',
)));
$wp_customize->add_setting('footer_color', array(
'default' => '#3388FF',
'sanitize_callback' => 'sanitize_hex_color',
));
$wp_customize->add_control(new WP_Customize_Color_Control($wp_customize, 'footer_color', array(
'label' => __('Footer Color', 'custom-theme'),
'section' => 'theme_colors',
)));
$wp_customize->add_setting('text_color', array(
'default' => '#333',
));
$wp_customize->add_control(new WP_Customize_Color_Control($wp_customize, 'text_color', array(
'label' => __('Text Color', 'custom-theme'),
'section' => 'theme_colors',
)));
}
add_action('customize_register', 'theme_customize_register');
In this code, we’ve added a setting and control for the primary color of the theme. The setting includes default color, a live preview, and a sanitize callback to ensure a valid color value. The control is added to the ‘colors’ section in the Customizer and labeled as ‘Primary Color.’
You can extend this concept to create theme options for various elements, such as fonts, header styles, or even custom text.
Saving and Retrieving Theme Options
Once you’ve created your custom theme options, it’s crucial to save and retrieve these options in your theme. To save them, you’ll use the get_theme_mod()
function. For example, to retrieve the primary color we created earlier:
$primary_color = get_theme_mod('primary_color', '#3498db');
With the options saved, you can use these values to apply styles to your theme. For instance, you can dynamically apply the selected primary color to your theme’s buttons, links, or other elements by using the retrieved value in your CSS.
By adding custom theme options through the WordPress Customizer, you provide users with the ability to personalize your theme according to their preferences. This enhances the flexibility and appeal of your WordPress theme, making it a more attractive choice for a broader audience.
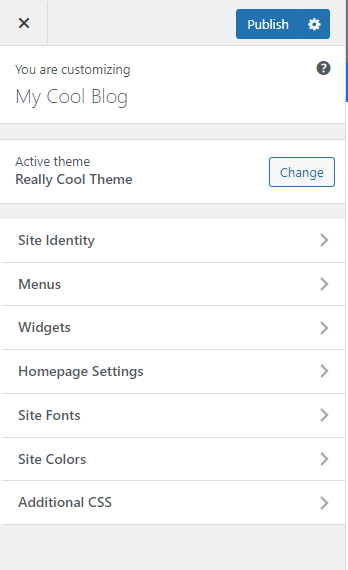
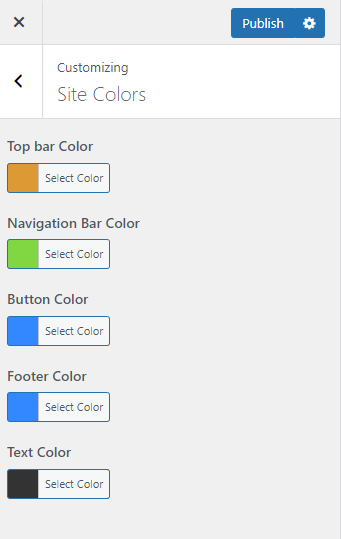
In our next installment, we’ll delve further into theme customization, exploring additional options and best practices to enhance your theme’s versatility and user experience. Stay tuned for more insights and knowledge-sharing in our ongoing journey through WordPress theme development.