In today's web development world, React has become one of the most popular libraries for building dynamic user interfaces. WordPress, on the other hand, remains one of the most widely used content management systems (CMS) for blogging, e-commerce, and more. Combining the flexibility of React with the powerful content management capabilities of WordPress can result in a highly dynamic and feature-rich website.
In this blog post, we’ll walk you through the process of integrating a React component into a WordPress theme. By the end of this tutorial, you will know how to set up a React app, embed a React component into a WordPress page, and ensure everything works seamlessly together.
If you're looking to create your very own WordPress theme, this comprehensive Guide to WordPress Theme Creation is the perfect starting point.
Step 1: Setting Up Your React Project
To get started, we first need to create a React application. Here are the steps you need to follow:
1.1 Install Node.js and npm
Before you begin, ensure that Node.js and npm (Node Package Manager) are installed on your machine. These tools are required for running a React application. If you don't have them installed yet, you can download the latest stable version of Node.js from the Node.js website. npm will be installed automatically with Node.js.
1.2 Create a New React Application
Once Node.js and npm are ready, you can create a new React app. Open your terminal and run the following command to create a new React app:
npx create-react-app react-wordpress-integration
This will set up a new React project in a folder called react-wordpress-integration
. It includes all the necessary dependencies and configuration files for a React application.
1.3 Navigate to the Project Directory
Next, go to the newly created project folder:
cd react-wordpress-integration
1.4 Run the React Application Locally
To test the React app and ensure everything is set up correctly, run:
npm start
The development server will start, and you can view your application by navigating to http://localhost:3000 in your browser. You should see the default React welcome page.
Step 2: Set Up Your WordPress Theme
Now that your React app is up and running, we need to prepare your WordPress theme to embed the React component. This involves editing the theme's index.php
file (or whereever you want to embed react component) and enqueuing the JavaScript file that will load the React app.
2.1 Add a Placeholder for the React Component
You can render a react component in any wordpress template file, but for simple demonstration, I will add a placeholder <div>
in index.php
where the React component will be rendered. WordPress uses PHP to generate HTML, and we’ll place the React component inside this container.
Open the index.php
file and add the following code:
<!DOCTYPE html>
<html <?php language_attributes(); ?>style="box-sizing: border-box;">
<head>
<meta charset="<?php bloginfo('charset'); ?>">
<meta name="viewport" content="width=device-width, initial-scale=1">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<title>React with Wordpress</title>
<?php wp_head(); ?>
</head>
<body <?php body_class(); ?>>
<main class="bg-white dark:bg-gray-950">
<p> This is my wordpress index.php file. </p>
<!-- Placeholder for React component -->
<div id="root"></div>
</main>
<?php wp_footer(); ?>
</body>
</html>
The id="root"
is the div
where your React app will be injected.
Step 3: Create the React Component
Let’s now create a simple React component to render within the WordPress site.
3.1 Create a React Component
Inside your React project folder (src
), create a folder called components
and create a new file HelloComponent.js
:
// src/components/tools/HelloComponent.js
import React from 'react';
const HelloComponent = () => {
return (
<div>
<h1>Hello, WordPress!</h1>
<p>This React component is successfully integrated with WordPress.</p>
</div>
);
};
export default HelloComponent;
This component is simple: it just displays a header and a paragraph to confirm that the integration has worked.
3.2 Update the index.js File
Next, open the src/index.js
file and update it to render the HelloComponent
we just created:
// src/index.js
import React from 'react';
import ReactDOM from 'react-dom/client';
import './index.css';
import HelloComponent from './components/tools/HelloComponent';
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(
<React.StrictMode>
<HelloComponent />
</React.StrictMode>
);
This code imports the HelloComponent
and renders it inside the root
element (the div we created in the WordPress theme).
To test, run:
npm start
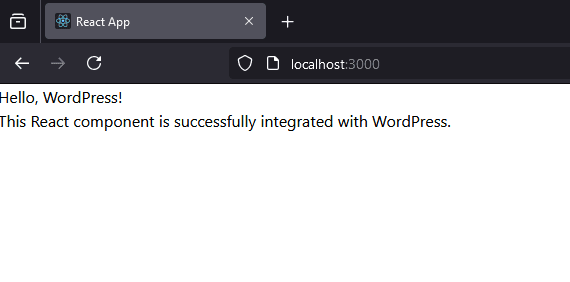
3.3 Build the React Application
Now that the React component is ready, you need to create a production build. Run the following command in your terminal:
npm run build
This command will create a build
folder with all the optimized assets, including JavaScript and CSS files.
3.4 Export the JavaScript File to WordPress
In the build/static/js/
folder, you will find the compiled main.<hash>.js
file. Copy this file to your WordPress theme’s /js/
folder (e.g., wp-content/themes/your-theme/js/
). I gave main.<hash>.js
a meaningful name, let's call it, react-app.js
.
If you want to include styles, you can copy the CSS file(s) from build/static/css/
to your theme as well.
Step 4: Enqueue the React App’s JavaScript File
Once you've built the React app, you'll need to enqueue the resulting JavaScript file into your WordPress theme. This can be done by modifying the functions.php
file.
In your theme’s functions.php
, add the following code to enqueue the React app:
function enqueue_react_app() {
// Register and enqueue the React app JavaScript file
wp_enqueue_script('react-app', get_template_directory_uri() . '/js/react-app.js', array(), null, true);
}
add_action('wp_enqueue_scripts', 'enqueue_react_app');
This code tells WordPress to include the react-app.js
file in your theme. You’ll later place this JavaScript file in the /js/
folder of your theme.
Step 5: Test the Integration Locally
Now it’s time to test the integration. Follow these steps:
5.1 Set Up WordPress Locally
You can use tools like Local by Flywheel or XAMPP to set up a local WordPress installation. Alternatively, you can use a staging or live WordPress site.
5.2 Activate the Theme
Log in to your WordPress admin dashboard, go to Appearance > Themes, and activate the theme that you’ve modified to include the React app.
5.3 Visit the WordPress Site
Now, visit your WordPress site in your browser. You should see the HelloComponent
rendered inside the div#root
placeholder. The text "Hello, WordPress!" should appear, confirming that the React component is working as expected.
Once everything is working, your React component will be successfully integrated into your WordPress theme.

Conclusion
In this tutorial, I've shown how to integrate a React component into a WordPress theme. By following these steps, you can enrich your WordPress site with dynamic, interactive features powered by React while continuing to manage your content through WordPress. This method allows you to combine the strengths of both WordPress and React, creating a modern and dynamic website experience for your visitors.
Feel free to experiment with more complex React components, such as forms, interactive UI elements, or even full-fledged apps, and enjoy the seamless combination of WordPress and React in your development projects.