
If you're looking to get started with GO language, this guide will help you to Set up GO Development Environment. From managing code files, packages, and modules, to working with dependencies and writing unit tests, I'll guide you through the basics of Go programming in a clear and concise way.
Introduction
GO is an open source, simple, secure and lightweight programming language originally designed and supported by Google. It has very impressive built-in support for concurrency, garbage collection, panic-recovery, network utilities etc. Coming from a C/C++ background I found it fairly easy to grasp.
Installation
GO installation is comparatively quicker. Go to this link https://go.dev/doc/install, chose your operating system, download and install.
A default installation path on windows OS is C:\Program Files\Go
Add Go to the Windows PATH Environment Variable:
- Search "Environment Variables" in the Windows search bar .
- In the Environment Variables window, under "System variables", find the "Path" variable and select it, then click on the "Edit" button.
- In the Edit Environment Variable window, click on the "New" button and add the path to the
bin
directory of GO, typically, that isC:\Program Files\Go\bin
. - Click "OK" to apply the changes.
After every installation, the first step is to ensure its successful completion through verification. Verify installation by
$ go
This simple go
command will return all possible options comes with go installation.
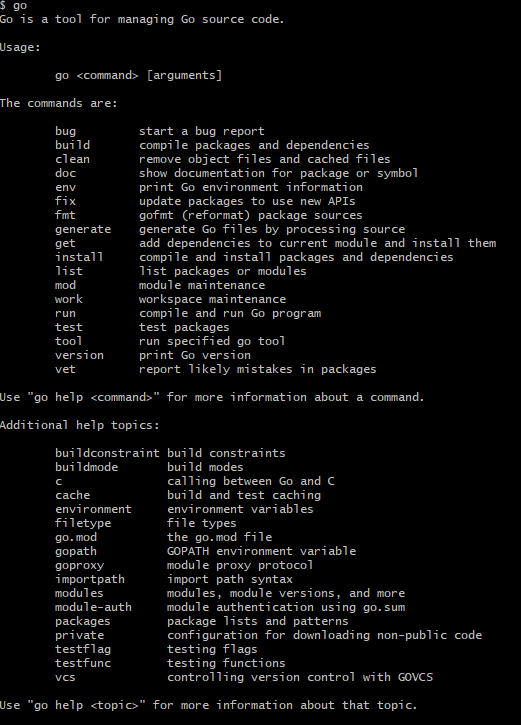
Setup environment variables
GO defines several environment variable, which can be displayed by
$ go env
We don’t need to know the purpose of all variables at the start, but GOROOT
and GOPATH
are the most important to understand in the beginning.
GOROOT
: points to the GO SDK installation directory.
Default value of GOROOT is C:\Program Files\Go
on Windows and at /usr/local/go/bin
on Linux and MAC OS. We often do not need to change this, unless you have some valid reason.
GOPATH
: is where GO looks for your source code or workspace.
Default value is %USERPROFILE%/go
on Windows and ~/go
on Linux and MACOS.
We need to add %USERPROFILE%/go
or ~/go
to the PATH variable.
Setting up environment variable value
$ go env -w GOROOT=/Go-root-path/
Unset environment variable
$ go env -w GOROOT
GO Playground
GO playground is an online service, which compiles, links and runs the source code. This is a very handy platform for a quick run.
IDE
GO is a lightweight programming language and many IDEs provide very good support, and plugins to help writing the code. I personally like to use Visual Studio Code and IntelliJ.
Hello World
So, we have set up environment to write our first HelloWolrd program in GO, open a text editor and write following code and save file with .go extension.
package main
import "fmt"
func main() {
fmt.Println("Hello world!")
}
Similar to other languages, GO arranges logically related files in the packages - in the first line we define our package main, which is the entry point of the programme.
fmt is a GO package to format the data structures like string, integer. We import fmt into our main() function and print the traditional “Hello World!” message.
Run this in terminal
$ go run main.go
Or in GO playground
GO directory structure
As written earlier, the default workspace location for GO is %USERPROFILE%/go
on Windows and ~/go
on Linux and MACOS, conventionally (but not necessarily) go workspace is structured as
~/
├─ src/
├─ pkg/
├─ bin/
src/
hosts the source code
pkg/
contains the compiled packages
bin/
consists of executables
Go Modules
Beginning with the classic 'Hello, world!' is always a good first step. However, as we write real-world applications, we require to add far more functionalities. This presents the need of arranging the code with increasing complexity, often those dependencies span across various packages and modules.
GO modules are the collection of related packages and are managed by the go.mod
file, which is used to specify the module import path and the dependencies.
Let’s create a new go module for our hello world program,
$ go mod init myrepo/first-go-app/hello-world
go mod init
command initializes a go module, in this example we name it as myrepo/first-go-app/hello-world
.
This will create a new go.mod
file
Add a package
Now we extend this example and add a package in our module. I add a simple json_handler
package which has only one function StringToStruct
in a single file. This function converts a json string into a struct.
package json_handler
import ( "encoding/json")
func StringToStruct(s string, i interface{}) error {
err := json.Unmarshal([]byte(s), i)
if err != nil {
return err
}
return nil
}
I want to call StringToStruct()
function defined in json_handler
package from my main
package file main.go
. I define a json string str
and send this to StringToObject()
function, which will convert this into an object of type Student
,
package main
import (
"myrepo/first-go-app/hello-world/json_handler"
"fmt"
)
var str = `{
"id": "1",
"name": "The User"
}`
type Student struct {
ID string `json:"id,omitempty"`
Name string `json:"name,omitempty"`
}
func main() {
var s Student
err := json_utils.StringToObject(str, &s)
if err != nil {
fmt.Printf("Something went wrong: %s", err.Error())
return
}
fmt.Println("ID = ", s.ID)
fmt.Println("Name = ", s.Name)
}
Running this code should print out
Unit Testing
GO provides a built-in support for unit test with very easy and quick setup - All you need to do is to,
- name your test file with suffix “_test.go”, such as
helloworld_test.go
- Import GO
testing
package - Start test function name by keyword Test like
TestMyFunction()
orTest_Myfunction()
- Pass go
testing
object in Test function, Like
go.mod
file will be like,
module myrepo/first-go-app/hello-world
go 1.19
require ( github.com/stretchr/testify v1.7.0 go.uber.org/zap v1.16.0)
and test file will have unit test function as,
func Test_StringToObject_Success(t *testing.T) {
}
- You would like to verify test results by asserting the tested function result. For that I have imported
github.com/stretchr/testify
.
Following is a complete unit test,
package json_handler_test
import (
"testing"
"github.com/stretchr/testify/assert"
)
var str = `{
"id": "1",
"name": "The User"
}`
type Student struct {
ID string `json:"id,omitempty"`
Name string `json:"name,omitempty"`
}
func Test_StringToObject_Success(t *testing.T) {
assertThat := assert.New(t)
var s Student
err := StringToObject(str, &s)
assertThat.Nil(err)
assertThat.Equal(s.ID, "1")
assertThat.Equal(s.Name, "The User")
}
Before running the code we should do
$ go mod tidy
$ go mod vendor
to resolve the dependencies.
Now we can run the test by
$ go test ./…
./…
runs all the tests in current and sub directories.
Useful go commands:
go build
compiles or builds the source code but does not execute the program
go run
compiles and execute the program
go test
runs the tests
go fmt
formats source code
go install
compiles and install the packages
go get
download the packages
go lint
analyzes Go source code for potential errors
That is it!
Hope you have leanred about how to create your first GO programme, module, package, pull some dependencies and write a unit test.